JAVA/수업 복습
자바 공부기록 49 - Formatter
본이qq
2022. 5. 11. 19:09
● 날짜 서식 클래스 - SimpleDateFormat
1
2
3
4
5
6
7
|
public class CalendarClass03 {
public static void main(String[] args) {
Date today = new Date();
SimpleDateFormat sdf1 = new SimpleDateFormat("yyyy-MM-dd");
System.out.println( sdf1.format(today));
|
cs |
-> sdf1의 서식에 맞춰 "2022-05-11"이 출력됨
●
y : 년도를 표시할 자리수 문자(yyyy:2020 yy:20)
M : 월을 표시할 자리수 문자(MM:01~12 M:1~12)
d : 일을 표시하는 자리수 문자 (dd:01~31 d:1~31)
H : 시에 해당하는 자리수 문자(HH : 01~23 H:1~23)
h : 시에 해당하는 자리수 문자(hh : 01~12 h:1~12)
m : 분
s : 초
S : 밀리초
a : 오전/오후
1
2
3
4
5
6
7
8
9
10
|
public class CalendarClass03 {
public static void main(String[] args) {
sdf2 = new SimpleDateFormat("yy년 MM월 dd일 E요일");
sdf3 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
sdf4 = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss a");
System.out.println(sdf2.format(today));
System.out.println(sdf3.format(today));
System.out.println(sdf4.format(today));
|
cs |
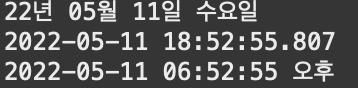
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
public class CalendarClass03 {
public static void main(String[] args) {
sdf5 = new SimpleDateFormat("오늘은 올 해의 D번째 날입니다");
sdf6 = new SimpleDateFormat("오늘은 이 달의 d번째 날입니다");
sdf7 = new SimpleDateFormat("오늘은 올 해의 w번째 주입니다");
sdf8 = new SimpleDateFormat("오늘은 이 달의 W번째 주입니다");
sdf9 = new SimpleDateFormat("오늘은 이 달의 F번째 E요일입니다");
System.out.println(sdf5.format(today));
System.out.println(sdf6.format(today));
System.out.println(sdf7.format(today));
System.out.println(sdf8.format(today));
System.out.println(sdf9.format(today));
|
cs |
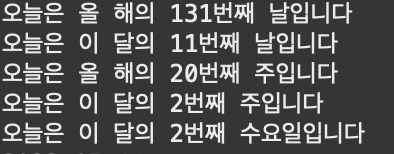
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public class CalendarClass03 {
public static void main(String[] args) {
Calendar cal = Calendar.getInstance();
Date day = cal.getTime(); //Calendar 객체의 현재 날짜값을 Date 값으로 변환
sdf1 = new SimpleDateFormat("yyyy-MM-dd");
sdf2 = new SimpleDateFormat("yyyy-MM-dd E요일");
sdf3 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
sdf4 = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss a");
System.out.println(sdf1.format(day)); //format(Date d)
System.out.println(sdf2.format(day));
System.out.println(sdf3.format(day));
System.out.println(sdf4.format(day));
|
cs |
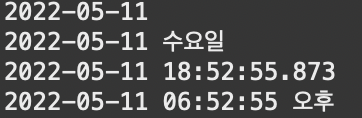
-SimpleDateFormat에는 parse메드를 이용하여 문자열을 날짜(Date)로 변환해주는 기능이 있습니다
다만 변환을 위해서는 설정된 서식을 반드시지켜야 하는 제약이 있습니다
1
2
3
4
5
6
7
8
9
10
11
|
public class CalendarClass03 {
public static void main(String[] args) throws ParseException {
String s2 = "2020/11/24";
SimpleDateFormat sdf1 = new SimpleDateFormat("yyyy/MM/dd");
Date d = sdf1.parse(s2);
SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy년 MM월 dd일");
System.out.println(sdf2.format(d));
|
cs |
● DecimalFormat -> 자릿수 서식
1
2
3
4
5
6
7
|
public class Formatter03 {
public static void main(String[] args) {
DecimalFormat df = new DecimalFormat("0000.00");
double number = 123.123456;
System.out.println(df.format(number));
|
cs |
-> 0123.12 출력
1
2
3
4
5
6
7
8
|
public class Formatter03 {
public static void main(String[] args) {
//천단위 구분기호 표시 패턴 0,000,000
DecimalFormat df = new DecimalFormat("0,000.00");
double number = 123456.123456;
System.out.println(df.format(number));
|
cs |
->123,456.12 출력
->다만, number에 89.123을 넣으면, 0,089.12가 출력됨
1
2
3
4
5
6
7
8
|
public class Formatter03 {
public static void main(String[] args) {
//무효의 0을 표시하지 않는 #을 0대신 사용
df = new DecimalFormat("#,###");
number = 123456.0;
System.out.println(df.format(number));
|
cs |
-> 123,456 출력
-> number에 89.123을 넣으면 89출력
1
2
3
4
5
6
7
8
|
public class Formatter03 {
public static void main(String[] args) {
//단위 또는 기호 첨가
df = new DecimalFormat("₩ #,###원");
number = 1234;
System.out.println(df.format(number));
|
cs |
-> ₩1,234원 출력